Array of Structures in C
Why use an array of structures?
Consider a case, where we need to store the data of 5 students. We can store it by using the structure as given below.
1.Grouping Related Data:
Structures allow you to group different data types under a single name. When you have related pieces of information (e.g., name, age, height for a person), it makes sense to group them together in a structure.
2. Sequential Access:
Array elements are stored in contiguous memory locations, making it easy to access them sequentially. This can lead to better cache locality and improved performance, especially when iterating through the array.
3. Simplified Code:
Using an array of structures can lead to more readable and maintainable code. Accessing and manipulating related data becomes more intuitive and organized compared to using separate arrays for each field.
4. Passing and Returning Data:
Functions can easily work with structured data by passing or returning entire structures or arrays of structures. This simplifies function interfaces and reduces the number of parameters.
5. Memory Efficiency:
Grouping related data together can reduce memory fragmentation. When structures are packed together in an array, there is less wasted space compared to having separate arrays for each data type.
6. Sorting and Searching:
Sorting and searching operations on arrays of structures are often more straightforward than dealing with multiple parallel arrays. You can define custom comparison functions that operate on the entire structure.
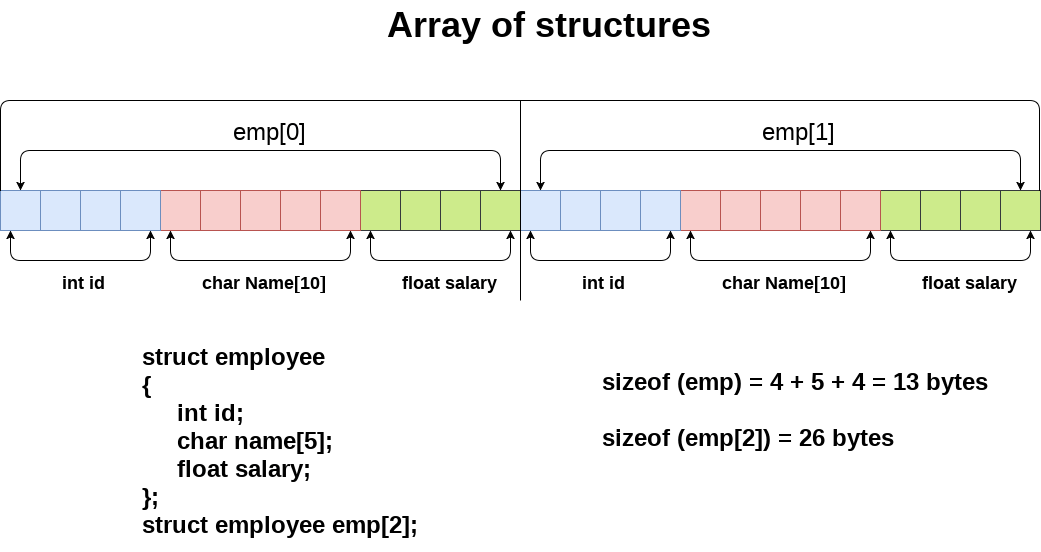
Let's see an example of an array of structures that stores information of 5 students and prints it
Example::
In this example, we define a structure Person with fields for name, age, and height. We then declare an array of Person structures called people with a size of 3. The program then prompts the user to enter details for three people, and finally, it displays the entered data.
You can adapt this example to your specific use case, adjusting the structure fields and array size accordingly.
7. Dynamic Memory Allocation:
When managing dynamic memory, having an array of structures simplifies memory allocation and deallocation. It's easier to allocate a block of memory for an array of structures than managing individual dynamic allocations for each field.
8. Code Readability:
The use of structures can improve code readability and maintainability by providing a natural way to represent real-world entities with their associated attributes.
Here's a simple example to illustrate the benefits:
In this example, an array of Point structures is used to represent 2D points, making the code more structured and easier to understand.
Here's a simple example to illustrate the benefits:
cstruct Point {
int x;
int y;
};
int main() {
struct Point points[3];
// Initialization
points[0].x = 1;
points[0].y = 2;
points[1].x = 3;
points[1].y = 4;
points[2].x = 5;
points[2].y = 6;
// Accessing and manipulating data
for (int i = 0; i < 3; ++i) {
printf("Point %d: x = %d, y = %d\n", i + 1, points[i].x, points[i].y);
}
return 0;
}
In this example, an array of Point structures is used to represent 2D points, making the code more structured and easier to understand.
Array of Structures in C
An array of structres in Ccan be defined as the collection of multiple structures variables where each variable contains information about different entities. The array of structures in C are used to store information about multiple entities of different data types. The array of structures is also known as the collection of structures.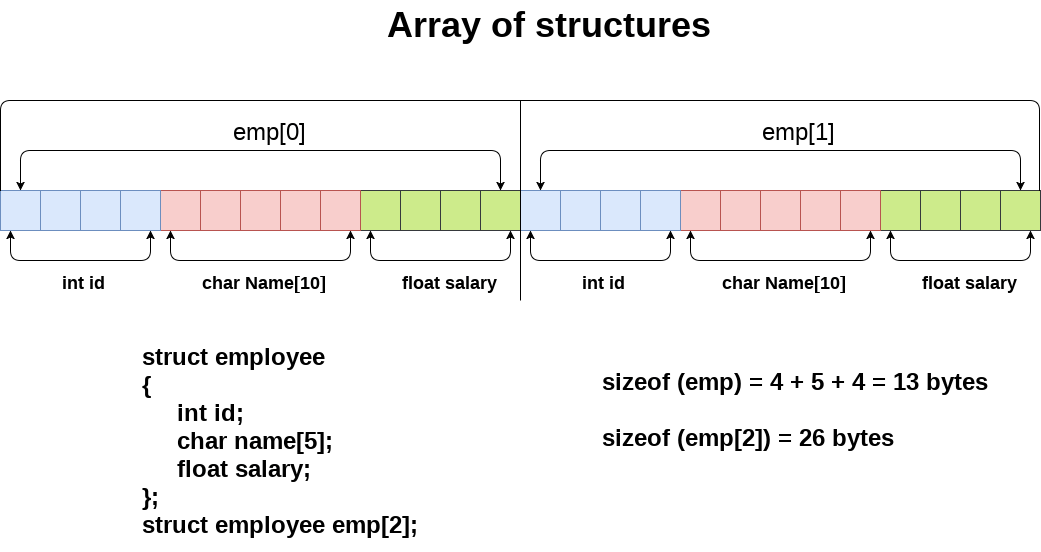
Let's see an example of an array of structures that stores information of 5 students and prints it
Example::
c#include <stdio.h>
// Define a structure
struct Person {
char name[50];
int age;
float height;
};
int main() {
// Declare an array of structures
struct Person people[3];
// Fill in data for each person
printf("Enter details for three people:\n");
for (int i = 0; i < 3; ++i) {
printf("Person %d:\n", i + 1);
printf("Name: ");
scanf("%s", people[i].name);
printf("Age: ");
scanf("%d", &people[i].age);
printf("Height: ");
scanf("%f", &people[i].height);
}
// Display the entered data
printf("\nDetails of the three people:\n");
for (int i = 0; i < 3; ++i) {
printf("Person %d:\n", i + 1);
printf("Name: %s\n", people[i].name);
printf("Age: %d\n", people[i].age);
printf("Height: %.2f\n", people[i].height);
printf("\n");
}
return 0;
}
In this example, we define a structure Person with fields for name, age, and height. We then declare an array of Person structures called people with a size of 3. The program then prompts the user to enter details for three people, and finally, it displays the entered data.
You can adapt this example to your specific use case, adjusting the structure fields and array size accordingly.
0 Comments